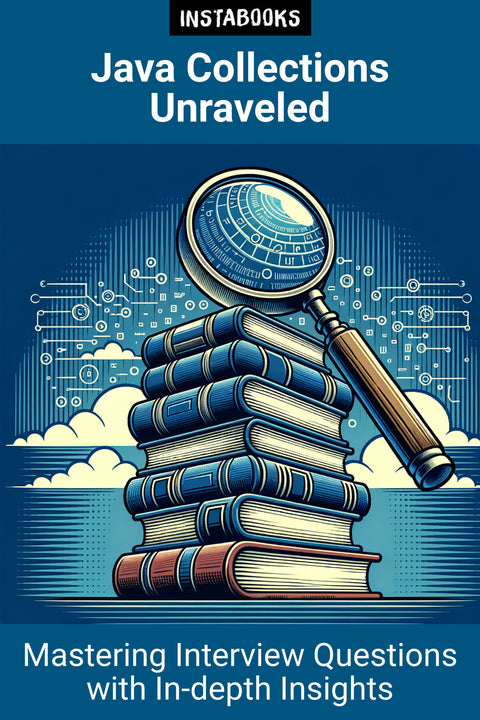
Instabooks AI (AI Author)
Java Collections Unraveled
Mastering Interview Questions with In-depth Insights
Premium AI Book (PDF/ePub) - 200+ pages
The journey into mastering Java Collections for interview success starts here! Java Collections Unraveled offers an authoritative guide through the complexities of the Collections framework.
Designed for a range of Java enthusiasts, from beginners to seasoned developers, this book delivers a comprehensive breakdown of data structures within Java's Collection API. The content is carefully structured to build understanding from the ground up, ensuring that readers are equipped with the knowledge needed to navigate interview questions confidently.
Expertly-Crafted Content for Every Java Developer
Chapter by chapter, each component of the Collections framework is dissected and reassembled to promote a robust comprehension. The book targets critical points that frequently appear in technical interviews, reinforced with real-world examples and exercises.
For those new to Java, you will find clear and concise explanations that illuminate core concepts without overwhelming details. On the flip side, veteran developers will appreciate the advanced topics and nuances that are essential for a successful interview performance.
Practical Applications and Lasting Knowledge
Insightful tricks and tips peppered throughout the chapters give you an edge over the competition. It's not just about knowing the right answers, but understanding the reasoning behind them. This book does not only prepare you for interviews but also enhances your coding expertise, ensuring long-term career development.
Embark on a learning journey with Java Collections Unraveled, and arm yourself with the wisdom required to excel. Turn interview challenges into opportunities as you confidently address complex topics with ease and expertise.
Table of Contents
1. Foundation of Java Collections- Introduction to the Collections Framework
- Core Interfaces and Implementations
- Iterable, Collection, and Iterator
2. Lists, Sets, and Queues Explained
- Differences and Usage Scenarios
- ArrayList vs LinkedList
- PriorityQueue and Deques
3. Navigating Maps and Trees
- Understanding HashMaps and TreeMap
- HashMap Internals and Performance
- NavigableMap and SortedMap
4. Concurrent Collections for Experts
- ConcurrentHashMap and its Operations
- CopyOnWriteArrayList and its Use Cases
- Synchronizers: CountDownLatch & CyclicBarrier
5. Comparators and Custom Ordering
- Implementing Comparators
- Ordering Collections
- Comparable vs Comparator
6. Algorithms and Collections Utilities
- Searching and Sorting
- Shuffling, Reversing, and Rotating
- Immutable Collections
7. Effective Use of Streams with Collections
- Stream API Basics
- Collection Operations and Stream
- Parallel Streams and Performance
8. Common Interview Questions Deconstructed
- Handling Collection-Based Problems
- Design Questions and Data Structure Choice
- Approaching Complex Algorithmic Challenges
9. Handling Collections in Practice
- Use Cases in Real-World Applications
- Memory Management and Optimization
- Best Practices for Collections
10. Advanced Topics in Java Collections
- Custom Collection Implementations
- Beyond the Standard Library
- Emerging Trends and Techniques
11. Developing Robust Java Systems
- Error Handling and Collections
- Scalability and Collection Management
- Secure Coding with Collections
12. Mock Interviews and Problem Solving
- Simulating Real Interview Scenarios
- Strategies for Effective Problem Solving
- Avoiding Common Pitfalls and Errors
How This Book Was Generated
This book is the result of our advanced AI text generator, meticulously crafted to deliver not just information but meaningful insights. By leveraging our AI book generator, cutting-edge models, and real-time research, we ensure each page reflects the most current and reliable knowledge. Our AI processes vast data with unmatched precision, producing over 200 pages of coherent, authoritative content. This isn’t just a collection of facts—it’s a thoughtfully crafted narrative, shaped by our technology, that engages the mind and resonates with the reader, offering a deep, trustworthy exploration of the subject.
Satisfaction Guaranteed: Try It Risk-Free
We invite you to try it out for yourself, backed by our no-questions-asked money-back guarantee. If you're not completely satisfied, we'll refund your purchase—no strings attached.